When it comes to text processing in Unix-like environments, awk and sed are two powerhouse tools that often confuse newcomers due to their overlapping capabilities. While both are designed to manipulate text, they serve distinct purposes and shine in different scenarios. Let’s break it down.
What is sed?
sed, short for “stream editor,” is a tool primarily used for editing text in a stream—line by line. It excels at simple substitutions, deletions, and insertions. Think of it as a find-and-replace wizard. For example, if you want to replace every instance of “cat” with “dog” in a file, sed is your go-to:
sed 's/cat/dog/g' file.txt
It’s lightweight, fast, and perfect for quick edits or batch processing via scripts. However, sed struggles with complex logic or multi-line operations—it’s more of a one-trick pony (albeit a very good one).
More complex sed examples
Insert a Line Before a Matching Pattern Across Multiple Files
Suppose you have several config files, and you want to insert a header line “# START CONFIG” before any line containing the word “server”. This also backs up the original files with a .bak extension:
sed -i.bak '/server/i # START CONFIG' *.conf
- -i.bak: Edits files in place and creates backups.
- /server/i: Inserts the specified text before lines matching “server”.
Replace Text Only on Lines Matching a Condition
Imagine a log file (logs.txt) where you want to replace “ERROR” with “ALERT” only on lines that also contain “2025”. This uses a conditional match:
sed '/2025/s/ERROR/ALERT/g' logs.txt
- /2025/: Targets only lines containing “2025”.
- s/ERROR/ALERT/g: Performs the substitution on those lines.
Delete Empty Lines and Lines Starting with a Comment, Then Number the Remaining Lines
For a script file (script.sh), remove blank lines and comments (lines starting with #), then prepend line numbers to what’s left:
sed '/^$/d; /^#/d; =;' script.sh | sed 'N;s/\n/ /'
- /^$/d: Deletes empty lines.
- /^#/d: Deletes lines starting with #.
- =: Adds line numbers (but on separate lines).
- The second sed combines the number and text into one line.
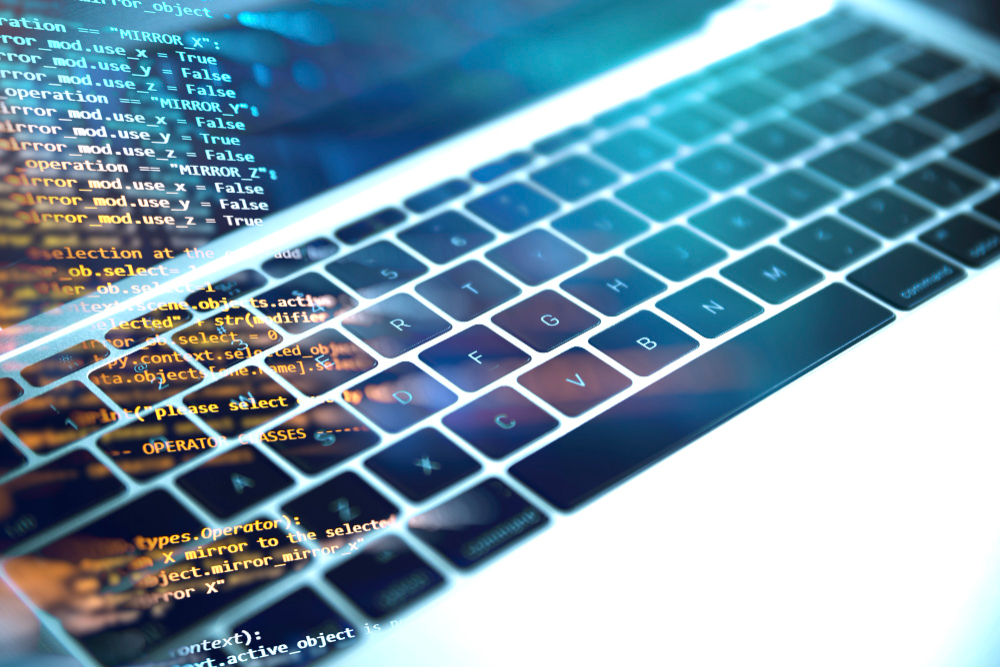
What is awk?
awk, named after its creators (Aho, Weinberger, and Kernighan), is a more versatile tool designed for text processing and data extraction. It’s essentially a mini programming language with built-in support for variables, conditionals, loops, and field-based parsing. If you need to extract specific columns from a CSV or perform calculations on data, awk shines. For instance, to print the second column of a space-separated file:
awk '{print $2}' file.txt
Unlike sed, awk treats input as structured data (fields and records), making it ideal for tasks requiring logic or analysis.
More complex awk examples
Sum Values in a Specific Column Based on a Condition
Given a CSV file (data.csv) with columns like name,age,score, calculate the total score for people over 30:
awk -F',' '$2 > 30 {sum += $3} END {print "Total score for age > 30:", sum}' data.csv
- -F’,’: Sets the field separator to a comma.
- $2 > 30: Checks if the age (second column) is over 30.
- sum += $3: Adds the score (third column) to a running total.
- END: Prints the result after processing all lines.
Extract and Reformat Matching Lines into a Custom Output
From a log file (access.log) with lines like 192.168.1.1 – GET /page 200, extract IP addresses and status codes for successful requests (status 200), reformatting them:
awk '$NF == 200 {print "IP: " $1 ", Status: " $NF}' access.log
- $NF == 200: Checks if the last field (number of fields) is “200”.
- print “IP: ” $1 “, Status: ” $NF: Prints the first field (IP) and last field (status) in a custom format.
Group and Count Occurrences by a Field, Sorting by Count
For a file (users.txt) with space-separated data like username dept role, count how many users are in each department and sort by count (descending):
awk '{dept[$2]++} END {for (d in dept) print d, dept[d]}' users.txt | sort -k2 -nr
- dept[$2]++: Increments a counter for each department (second field).
- END {for (d in dept) print d, dept[d]}: Prints each department and its count.
- sort -k2 -nr: Sorts by the second column (count) numerically in reverse order.
Key Differences
- Purpose: sed is for editing text (substitutions, deletions), while awk is for processing and analyzing data.
- Complexity: sed handles simple, line-based edits; awk supports complex logic and field manipulation.
- Syntax: sed uses cryptic regex-based commands; awk offers a more readable, programmatic approach.
- Use Case: Use sed to tweak text (e.g., replace words), and awk to slice and dice structured data (e.g., sum a column).
When to Use Which?
- Use sed for quick substitutions or line deletions—like fixing typos across files.
- Use awk when you need to extract fields, filter data, or perform calculations—like analyzing logs or reports.
Can They Work Together?
Absolutely! Piping sed into awk (or vice versa) is a common trick. For example, you might use sed to clean up a file and then awk to analyze it:
sed 's/ */ /g' file.txt | awk '{print $3}'
These examples highlight sed’s strength in intricate text edits and awk’s power in data manipulation and logic.
Power Your Projects with vpszen.com VPS Solutions
Looking for reliable hosting to run your Linux servers and host your next big project? VpsZen.com has you covered with top-tier VPS options tailored to your needs.
Choose from ARM64 VPS Servers for energy-efficient performance, or Root VPS Servers for virtual servers with dedicated resources.